Learning Go quickly (WIP)
Learning the paradigms, getting up and running quickly
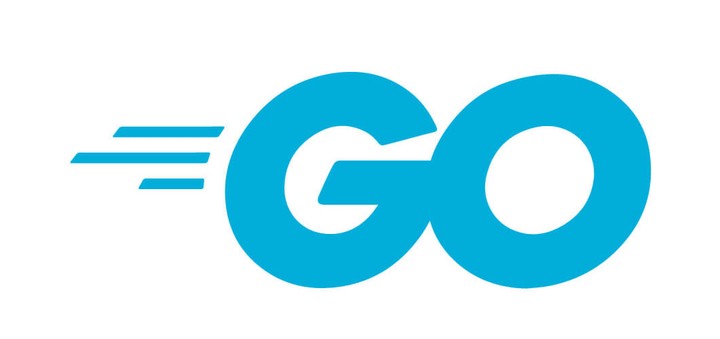
Introduction
My employer uses Go as their backend language. I’ve heard good things about Go from people I respect, so this is a great time to try it out!
I asked my friend Dewald for some resources to get up to speed quickly. Here’s what he sent me:
I decided to go with the official the official website, and to follow the examples there.
This post will be a useful reference for myself (and maybe others) - my notes on learning a new language as an experienced engineer.
The GitHub repo for this post is here.
This is a draft that I will update as I learn more.
Running Go code
Run the command go run <filename>.go
to run a Go program.
Structure of a Go program
- A Go program is made up of packages. Programs start running in package
main
. - Names are only exported if they begin with a capital letter. This makes them accessible outside the package they are defined in.
File names
Info taken from this Stack Overflow post
- File names that begin with “.” or “_” are ignored by the go tool
- Files with the suffix _test.go are only compiled and run by the go test tool.
- Files with os and architecture specific suffixes automatically follow those same constraints, e.g. name_linux.go will only build on linux, name_amd64.go will only build on amd64.
- File names should match the package name.
Functions
If a function takes many arguments of the same type, you can omit the type until the last argument.
func add_many(x, y, z, a, b, c int) int { return x + y + z + a + b + c }
A function can return any number of results.
func add_two(x, y, z int) (int, int, int, string) { return x + 2, y + 2, z + 2, "Finished adding 2" }
You can name return values. Also note the naked return here (I don’t like this naked return, but it’s a thing in Go). Note the way this that function is called with the return values
x
andy
being set.func split(sum int) (x, y int) { x = sum * 4 / 9 y = sum - x return } x, y := split(17)
Types
You can interpolate the type and value of a variable like this
fmt.Printf("Type: %T, Value: %v\n", check_my_type, check_my_type)
Flow control
This is pretty cool. In this example, v
is only in scope during the if
statement (or related else
statement).
func pow(x, n, lim float64) float64 {
if v := math.Pow(x, n); v < lim {
return v
}
return lim
}
Defer and panic
defer
schedules a function call to be run after the current function scope completes.panic
stops the normal execution of a function and runs any deferred functions.- It’s like throwing an exception in other languages.
- Dewald comments that it is “highly discouraged in production code.”
- There is also a
recover
keyword which is likecatch
to recover from apanic
state. - If you run
os.Exit
orlog.Fatal
, yourdefer
functions will not run.
If you run the code below:
for i := 0; i < 10; i++ {
defer fmt.Println(i)
if i == 5 {
panic("Exiting early to see how defer runs after a panic")
}
}
You will see the following output:
5
4
3
2
1
0
World
panic: Exiting early to see how defer runs after a panic
goroutine 1 [running]:
main.main()
/learning-go/FlowControl/flow_control.go:71 +0x72f
exit status 2